Sharing Our Passion for Technology
& Continuous Learning
Unlearning jQuery
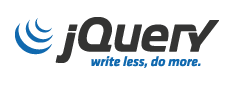
Now that you have already learned jQuery, let's unlearn it and get back to the basics. In this article, we will quickly go over the most common things that jQuery is currently used for and convert them to pure javascript.
jQuery is a great tool and contains polyfills that are beneficial. However, more often than not, I see it being abused and used for everything just for the sake of code simplicity. For example a webpage that is only using some basic javascript might not need jQuery at all, but a better understanding of javascript. Let's take some of the basic things I have seen and convert them directly to javascript, in order to unlearn jQuery.
Selectors
Starting from the basics, we have thejQuery selector $(“#box”)
depending on what we want to do with the result, the jQuery element, it might just be better to write it in javascript. A proper time to use the jQuery selector would be if you plan on calling a jQuery plugin, polyfill jquery functions, etc. More often than not, I simply see the selector and then basic calls to .attr(),.addClass(), or something similar. As you should already know, a similar translation to get the element by id would be as follows: document.getElementById(“box”)
. Check out the speed tests. The basic reason for this is because you're not pulling in all of the jQuery overhead.
Selectors don't have to stop with just getElementById
. As you know, there is also getElementByClass
and modern browsers even have querySelector. Sometimes jQuery selectors are much more complex and look something like $(“#box iframe”)
. So how do I get the iframe in order to change the source of it without using jQuery? This answer would depend on how your html has been setup. In this case, it looks like the iframe does not have an id or a class to select by, so we could use the querySelector to do a direct translation querySelector(“#box iframe”)
. But wait, that was too easy, and maybe not the fastest way. As we take a closer look at the HTML we know that the iframe is always the second child of #box. Since we now know vital information like this, we can simply:
document.getElementById(“box”).childNodes[1]
. If you would like to visit a speed test for this, please view here. Anyway, there are lots of way to do it, and the easiest might not always be the fastest.
Attributes
From the example above, we forgot to change the source of the iframe. Well let's take a look into that! Your code might have something like$(“#box iframe”).attr(‘src’,’some-url’)
. In order to change the source, it is even easier than using jQuery since we have direct access to the src attribute. Let's look at an example: var foo = document.querySelector(“#box iframe”).src = “some-url”
. That will do it! But the example did not let you access the attributes, something you might be wondering. Let's say you have an attribute called “data-lazyload”. That is simply just: foo.getAttribute(“data-lazyload”)
.
Setting up and removing class names are also something that I see jQuery used for where it does not necessarily need to be. Maybe you have something like $(“#box”).addClass(“loaded”)
. Then something: document.getElementById(“box”).className = “loaded”
might work for your needs. There is only one thing to think about when using className and not jQuery’s addClass; that is that you're assigning className a specific class and not actually appending the class to the selected element, so using the above could override your existing changes.
There is also a bunch of other stuff like replacing $(“#box”).css(“z-index”,”10”)
with document.getElementById(“box”).style.zIndex = “10”.
However, I am not a huge fan of inline styles so you're more than welcome to simply take note of the above.
Ajax
We have now covered all of the basic, the unlearning of jQuery to solve your everyday needs. There is now just one more that I want to leave you with, and that is AJAX. In today's world there are several times where we need to interact with REST services. In jQuery we might do:$.get(“/services/loggedin”,function(e) { $(“#box”).addClass(“user-loggedin”); })
. In a modern browser we could simply use XMLHttpRequest. It would look something like this:
var http = new XMLHttpRequest();
http.open(“GET”,”/services/loggedin”, true);
http.onload = function() { document.getElementById(“box”).className = “user-loggedin” };
http.send();
The above is all self explanatory except perhaps not the true, at the end of the http.open. This basically says if the call should be async or not. Since default jQuery $.get is async we set it to true in the above example.